5.13 Temperature - Humidity¶
The DHT11 is a temperature and humidity sensor commonly used for environmental measurements. It is a digital sensor that communicates with a microcontroller to provide temperature and humidity readings.
In this project, we will be reading the DHT11 sensor and printing out the temperature and humidity values it detects.
By reading the data provided by the sensor, we can obtain the current temperature and humidity values in the environment. These values can be used for real-time monitoring of environmental conditions, weather observations, indoor climate control, humidity reports, and more.
Required Components
In this project, we need the following components.
It’s definitely convenient to buy a whole kit, here’s the link:
Name |
ITEMS IN THIS KIT |
LINK |
---|---|---|
ESP32 Starter Kit |
320+ |
You can also buy them separately from the links below.
COMPONENT INTRODUCTION |
PURCHASE LINK |
---|---|
- |
|
Available Pins
Here is a list of available pins on the ESP32 board for this project.
Available Pins
IO13, IO12, IO14, IO27, IO26, IO25, IO33, IO15, IO2, IO0, IO4, IO5, IO18, IO19, IO21, IO22, IO23
Schematic
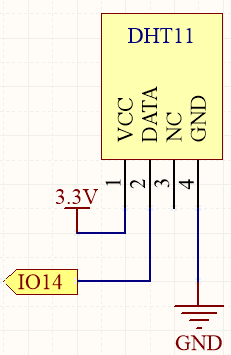
Wiring
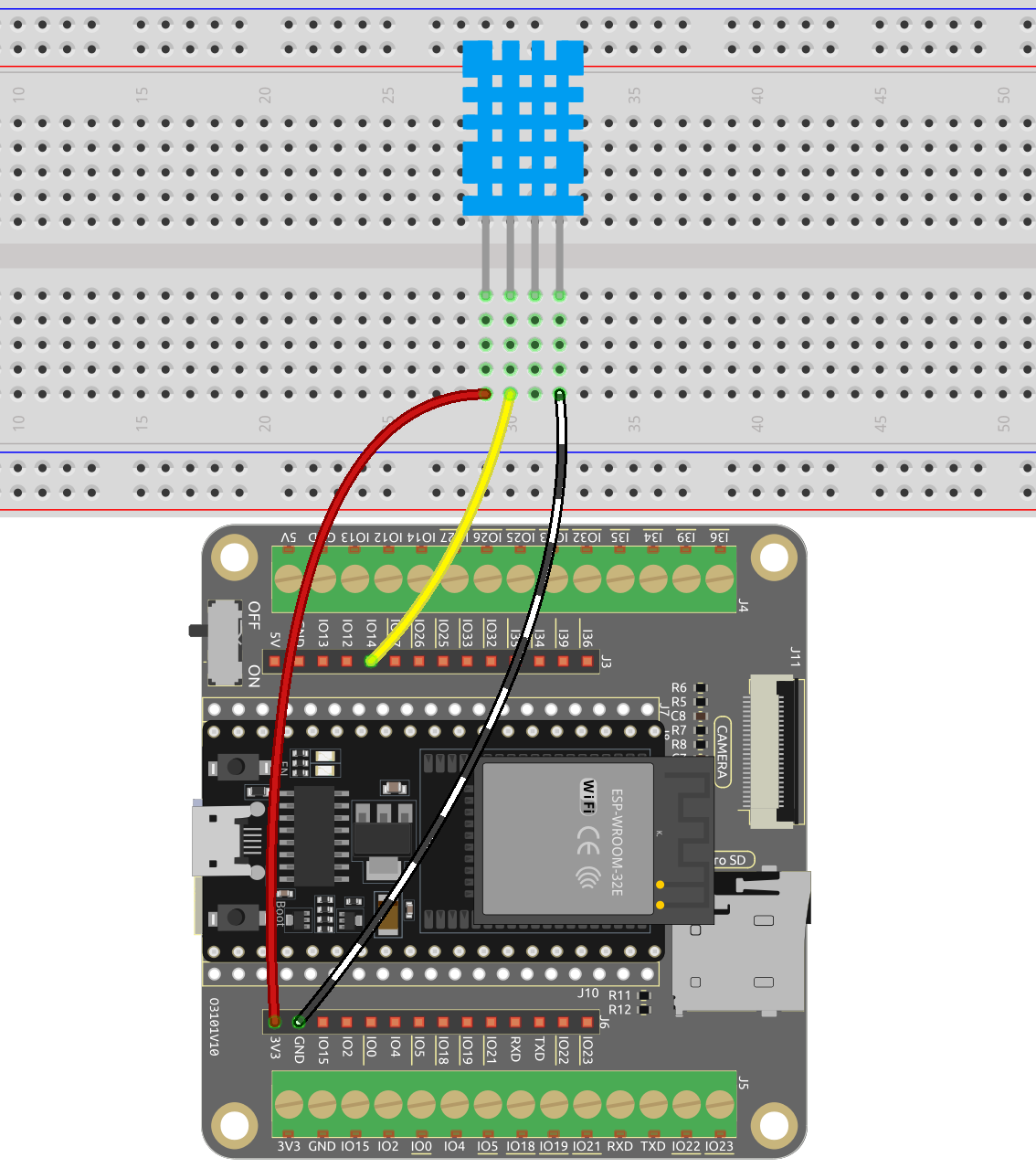
Code
Note
Open the
5.13_dht11.ino
file under the path ofesp32-starter-kit-main\c\codes\5.13_dht11
.After selecting the board (ESP32 Dev Module) and the appropriate port, click the Upload button.
The
DHT sensor library
library is used here, you can install it from the Library Manager.
After the code is uploaded successfully, you will see the Serial Monitor continuously print out the temperature and humidity, and as the program runs steadily, these two values will become more and more accurate.
How it works?
Includes the
DHT.h
library, which provides functions to interact with the DHT sensors. Then, set the pin and type for the DHT sensor.#include "DHT.h" #define DHTPIN 14 // Set the pin connected to the DHT11 data pin #define DHTTYPE DHT11 // DHT 11 DHT dht(DHTPIN, DHTTYPE);
Initializes serial communication at a baud rate of 115200 and initializes the DHT sensor.
void setup() { Serial.begin(115200); Serial.println("DHT11 test!"); dht.begin(); }
In the
loop()
function, read temperature and humidity values from the DHT11 sensor, and print them to the serial monitor.void loop() { // Wait a few seconds between measurements. delay(2000); // Reading temperature or humidity takes about 250 milliseconds! // Sensor readings may also be up to 2 seconds 'old' (it's a very slow sensor) float humidity = dht.readHumidity(); // Read temperature as Celsius (the default) float temperture = dht.readTemperature(); // Check if any reads failed and exit early (to try again). if (isnan(humidity) || isnan(temperture)) { Serial.println("Failed to read from DHT sensor!"); return; } // Print the humidity and temperature Serial.print("Humidity: "); Serial.print(humidity); Serial.print(" %\t"); Serial.print("Temperature: "); Serial.print(temperture); Serial.println(" *C"); }
The
dht.readHumidity()
function is called to read the humidity value from the DHT sensor.The
dht.readTemperature()
function is called to read the temperature value from the DHT sensor.The
isnan()
function is used to check if the readings are valid. If either the humidity or temperature value is NaN (not a number), it indicates a failed reading from the sensor, and an error message is printed.
Learn More
You can also display the temperature and humidity on the I2C LCD1602.
Note
You can open the file
5.10_thermistor_lcd.ino
under the path ofeuler-kit/arduino/5.10_thermistor_lcd
.After selecting the board (ESP32 Dev Module) and the appropriate port, click the Upload button.
The
LiquidCrystal_I2C
andDHT sensor library
libraries are used here, you can install them from the Library Manager.