Note
Hello, welcome to the SunFounder Raspberry Pi & Arduino & ESP32 Enthusiasts Community on Facebook! Dive deeper into Raspberry Pi, Arduino, and ESP32 with fellow enthusiasts.
Why Join?
Expert Support: Solve post-sale issues and technical challenges with help from our community and team.
Learn & Share: Exchange tips and tutorials to enhance your skills.
Exclusive Previews: Get early access to new product announcements and sneak peeks.
Special Discounts: Enjoy exclusive discounts on our newest products.
Festive Promotions and Giveaways: Take part in giveaways and holiday promotions.
👉 Ready to explore and create with us? Click [here] and join today!
2.2.3 Reed Switch Module¶
Introduction¶
In this project, we will learn about the reed switch, which is an electrical switch that operates by means of an applied magnetic field.
Required Components¶
In this project, we need the following components.
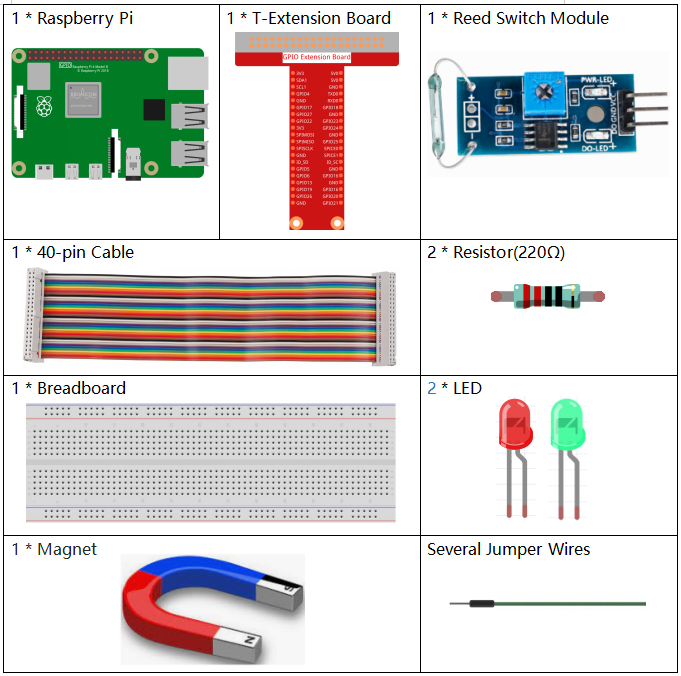
It’s definitely convenient to buy a whole kit, here’s the link:
Name |
ITEMS IN THIS KIT |
LINK |
---|---|---|
Raphael Kit |
337 |
You can also buy them separately from the links below.
COMPONENT INTRODUCTION |
PURCHASE LINK |
---|---|
Schematic Diagram¶
T-Board Name |
physical |
wiringPi |
BCM |
GPIO17 |
Pin 11 |
0 |
17 |
GPIO27 |
Pin 13 |
2 |
27 |
GPIO22 |
Pin 15 |
3 |
22 |
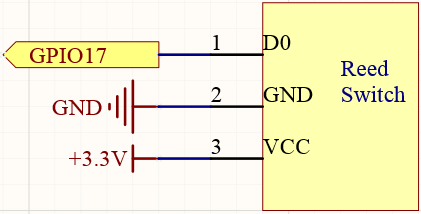
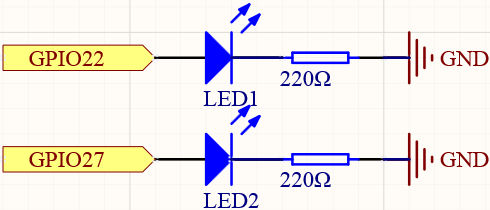
Experimental Procedures¶
Step 1: Build the circuit.
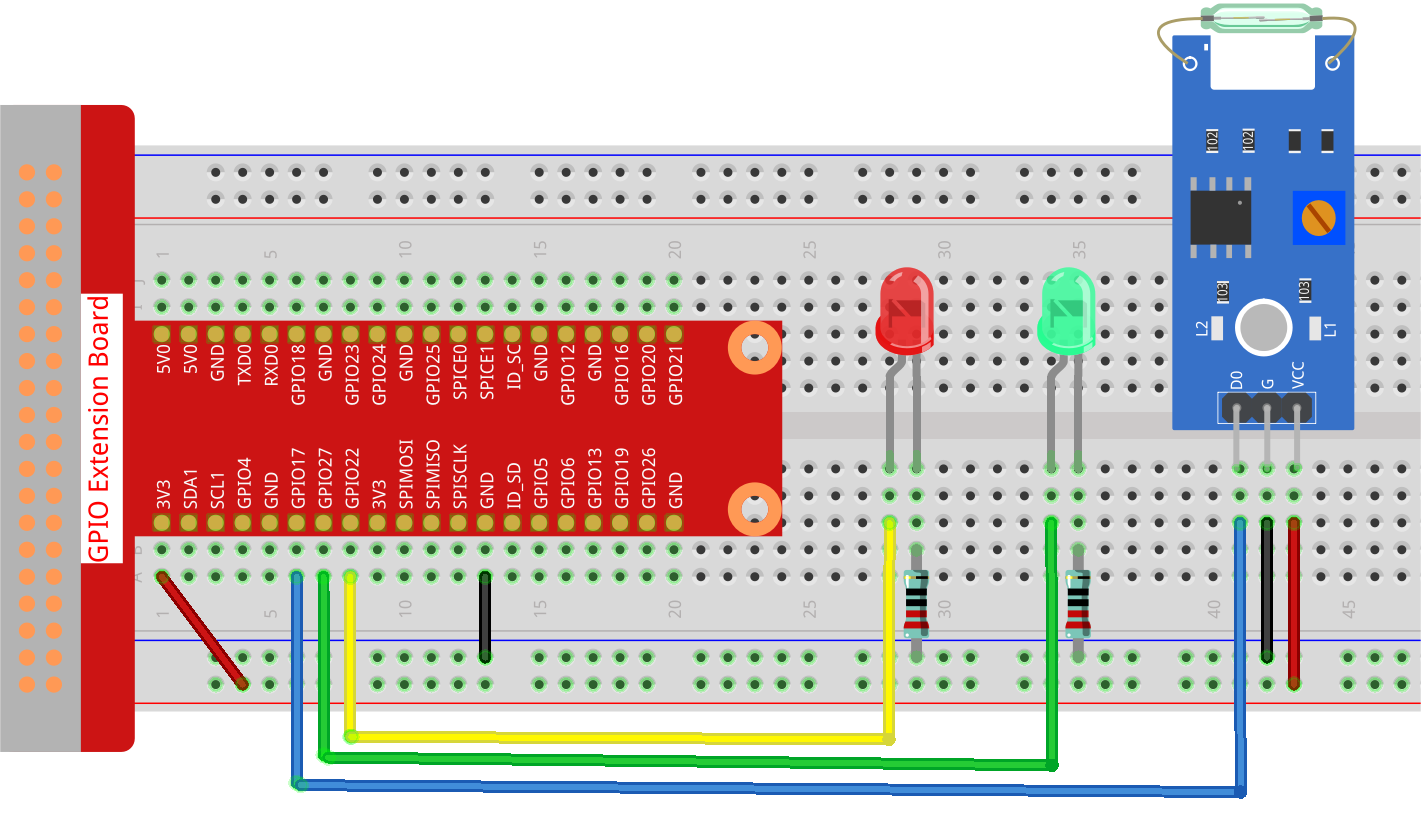
Step 2: Change directory.
cd ~/raphael-kit/python-pi5
Step 3: Run.
sudo python3 2.2.4_ReedSwitch_zero.py
The green LED will light up when the code is run. If a magnet is placed close to the reed switch module, the red LED lights up; take away the magnet and the green LED lights up again.
Code
Note
You can Modify/Reset/Copy/Run/Stop the code below. But before that, you need to go to source code path like raphael-kit/python-pi5
. After modifying the code, you can run it directly to see the effect.
#!/usr/bin/env python3
from gpiozero import LED, Button
# Initialize the reed switch and LEDs using GPIO Zero
reed_switch = Button(17, pull_up=True) # Reed switch on GPIO 17, using an internal pull-up resistor
green_led = LED(27) # Green LED connected to GPIO pin 27
red_led = LED(22) # Red LED connected to GPIO pin 22
def update_leds():
"""
Update the state of the LEDs based on the reed switch.
Turns the red LED on and green LED off when the reed switch is pressed, and vice versa.
"""
if reed_switch.is_pressed:
green_led.off() # Turn off the green LED
red_led.on() # Turn on the red LED
else:
green_led.on() # Turn on the green LED
red_led.off() # Turn off the red LED
try:
green_led.on() # Turn on the green LED at the start
while True:
# Set the callback functions for reed switch state changes
reed_switch.when_pressed = update_leds # Callback when the switch is pressed
reed_switch.when_released = update_leds # Callback when the switch is released
except KeyboardInterrupt:
# Clean up resources and exit on Ctrl+C
green_led.off()
red_led.off()
pass
Code Explanation
This line specifies that the script is to be run using Python 3. It imports
LED
andButton
(used for the reed switch) from the gpiozero library.#!/usr/bin/env python3 from gpiozero import LED, Button
Initializes the reed switch on GPIO pin 17 with an internal pull-up resistor. Also initializes two LEDs connected to GPIO pins 27 and 22.
# Initialize the reed switch and LEDs using GPIO Zero reed_switch = Button(17, pull_up=True) # Reed switch on GPIO 17, using an internal pull-up resistor green_led = LED(27) # Green LED connected to GPIO pin 27 red_led = LED(22) # Red LED connected to GPIO pin 22
Defines the
update_leds
function, which updates the LED states based on the reed switch’s state. The red LED is turned on and the green LED is turned off when the switch is pressed, and the opposite when released.def update_leds(): if reed_switch.is_pressed: green_led.off() # Turn off the green LED red_led.on() # Turn on the red LED else: green_led.on() # Turn on the green LED red_led.off() # Turn off the red LED
Sets the initial state of the green LED to on. The main loop assigns the
update_leds
function as callbacks for thewhen_pressed
andwhen_released
events of the reed switch. Includes exception handling for KeyboardInterrupt to clean up and exit the program gracefully.try: green_led.on() # Turn on the green LED at the start while True: # Set the callback functions for reed switch state changes reed_switch.when_pressed = update_leds # Callback when the switch is pressed reed_switch.when_released = update_leds # Callback when the switch is released except KeyboardInterrupt: # Clean up resources and exit on Ctrl+C green_led.off() red_led.off() pass