Access Control System¶
The primary function of this code is to perform user authentication using an RFID module. If the authentication is successful, it controls a stepper motor to open the door and emits a sound through a buzzer to indicate the authentication result. If the authentication fails, the door will not open.
You can open the serial monitor to view the ID of your RFID card and re-config the password in this code.
Required Components
In this project, we need the following components.
It’s definitely convenient to buy a whole kit, here’s the link:
Name |
ITEMS IN THIS KIT |
LINK |
---|---|---|
Elite Explorer Kit |
300+ |
You can also buy them separately from the links below.
COMPONENT INTRODUCTION |
PURCHASE LINK |
---|---|
- |
|
- |
|
- |
Wiring
Note
To protect the Power Supply Module’s battery, please fully charge it before using it for the first time.
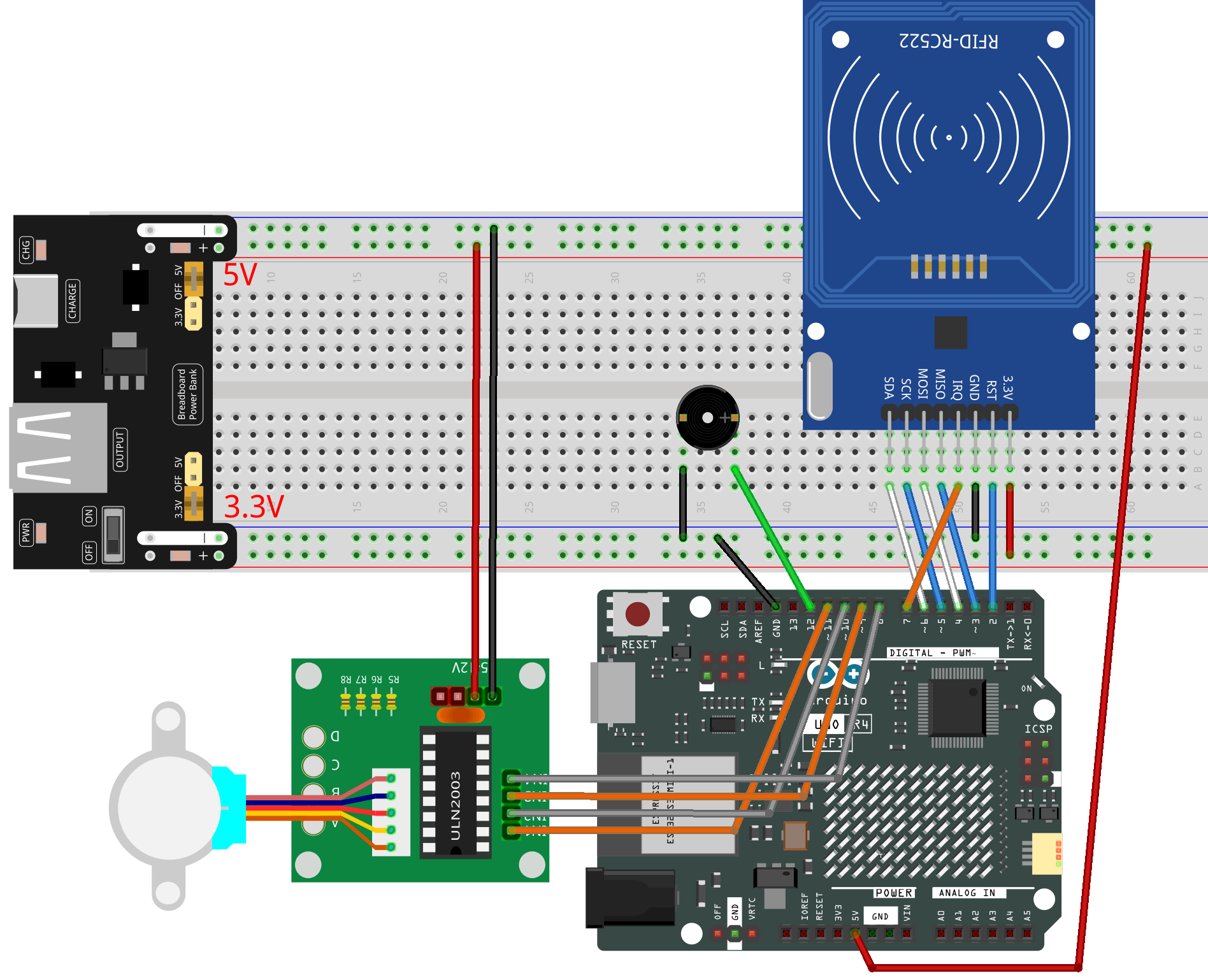
Schematic
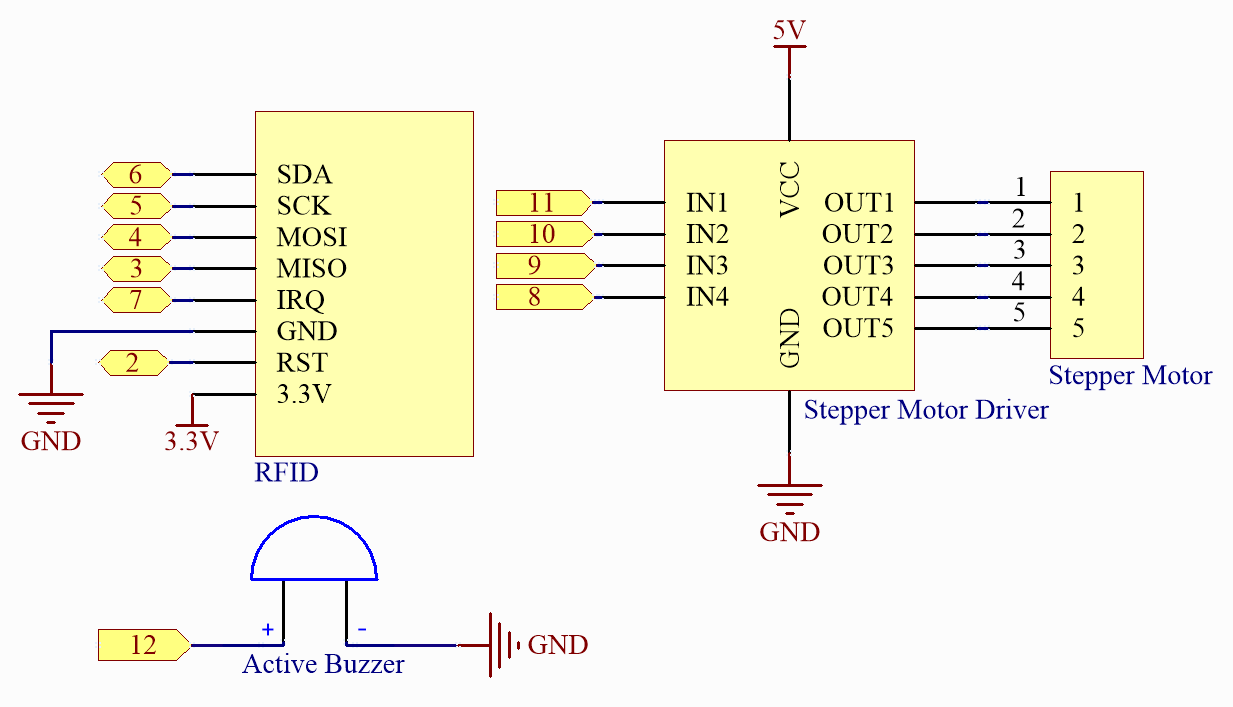
Code
Note
You can open the file
09_access_control_system.ino
under the path ofelite-explorer-kit-main\fun_project09_access_control_system
directly.Or copy this code into Arduino IDE.
Note
The
RFID1
library is used here. The library can be found in theelite-explorer-kit-main/library/
directory, or you can click hereRFID1.zip
to download it. Refer to Manual Installation for a tutorial on how to install it.
How it works?
Below is a step-by-step explanation of the code:
Include Library Files: You have included three library files:
rfid1.h
,Stepper.h
, andWire.h
. These libraries are used to communicate with the RFID module, stepper motor, and for I2C communication.Constant Definitions: You have defined some constants, including
ID_LEN
(length of the ID),stepsPerRevolution
(steps per revolution for the stepper motor),rolePerMinute
(stepper motor’s speed), as well as the four stepper motor pins (IN1, IN2, IN3, IN4), buzzer pin (buzPin
), and variables related to authentication.Variable Definitions: You’ve defined variables such as an array to store the read user ID (
userIdRead
), authenticated user ID (userId
), and a boolean variable (approved
) to indicate successful authentication.Object Instantiation: You’ve created instances of two objects:
RFID1 rfid
andStepper stepper
for interacting with the RFID module and stepper motor, respectively.setup()
: In thesetup()
function, you initialize the stepper motor’s speed, set the buzzer pin as an output, and initialize the RFID module.loop()
: In theloop()
function, your main logic runs. Ifapproved
is 0 (indicating not authenticated yet), it calls therfidRead()
function to read data from the RFID module and then clears theuserIdRead
array. Ifapproved
is 1 (indicating successful authentication), it calls theopenDoor()
function to open the door and resetsapproved
to 0.beep()
: This function controls the buzzer sound based on theduration
andfrequency
parameters provided.verifyPrint()
: This function produces different buzzer sounds based on theresult
parameter to indicate whether authentication was successful.openDoor()
: This function controls the stepper motor to open the door to a certain angle (doorStep
) and then waits for a period before closing the door.rfidRead()
: This function reads data from the RFID module, first callinggetId()
to get the user ID and thenidVerify()
to verify if the user ID matches the authenticated ID.getId()
: This function retrieves the user ID from the RFID module and stores it in theuserIdRead
array. It emits a beep if reading fails.idVerify()
: This function verifies if the user ID matches the authenticated ID and produces a sound indicating successful or failed authentication.