11. Video CarΒΆ
This program will provide a First Person View from the PiCar-X! Use the keyboards WSAD keys to control the direction of movement, and the O and P to adjust the speed.
Run the Code
cd ~/picar-x/example
sudo python3 11.video_car.py
Once the code is running, you can see what PiCar-X is shooting and control it by pressing the following keys.
O: speed up
P: speed down
W: forward
S: backward
A: turn left
D: turn right
F: stop
T: take photo
Ctrl+C: quit
View the Image
After the code runs, the terminal will display the following prompt:
No desktop !
* Serving Flask app "vilib.vilib" (lazy loading)
* Environment: production
WARNING: Do not use the development server in a production environment.
Use a production WSGI server instead.
* Debug mode: off
* Running on http://0.0.0.0:9000/ (Press CTRL+C to quit)
Then you can enter http://<your IP>:9000/mjpg
in the browser to view the video screen. such as: https://192.168.18.113:9000/mjpg
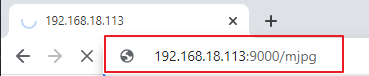
code
#!/usr/bin/env python3
from robot_hat.utils import reset_mcu
from picarx import Picarx
from vilib import Vilib
from time import sleep, time, strftime, localtime
import readchar
import os
user = os.getlogin()
user_home = os.path.expanduser(f'~{user}')
reset_mcu()
sleep(0.2)
manual = '''
Press key to call the function(non-case sensitive):
O: speed up
P: speed down
W: forward
S: backward
A: turn left
D: turn right
F: stop
T: take photo
Ctrl+C: quit
'''
px = Picarx()
def take_photo():
_time = strftime('%Y-%m-%d-%H-%M-%S',localtime(time()))
name = 'photo_%s'%_time
path = f"{user_home}/Pictures/picar-x/"
Vilib.take_photo(name, path)
print('\nphoto save as %s%s.jpg'%(path,name))
def move(operate:str, speed):
if operate == 'stop':
px.stop()
else:
if operate == 'forward':
px.set_dir_servo_angle(0)
px.forward(speed)
elif operate == 'backward':
px.set_dir_servo_angle(0)
px.backward(speed)
elif operate == 'turn left':
px.set_dir_servo_angle(-30)
px.forward(speed)
elif operate == 'turn right':
px.set_dir_servo_angle(30)
px.forward(speed)
def main():
speed = 0
status = 'stop'
Vilib.camera_start(vflip=False,hflip=False)
Vilib.display(local=True,web=True)
sleep(2) # wait for startup
print(manual)
while True:
print("\rstatus: %s , speed: %s "%(status, speed), end='', flush=True)
# readkey
key = readchar.readkey().lower()
# operation
if key in ('wsadfop'):
# throttle
if key == 'o':
if speed <=90:
speed += 10
elif key == 'p':
if speed >=10:
speed -= 10
if speed == 0:
status = 'stop'
# direction
elif key in ('wsad'):
if speed == 0:
speed = 10
if key == 'w':
# Speed limit when reversing,avoid instantaneous current too large
if status != 'forward' and speed > 60:
speed = 60
status = 'forward'
elif key == 'a':
status = 'turn left'
elif key == 's':
if status != 'backward' and speed > 60: # Speed limit when reversing
speed = 60
status = 'backward'
elif key == 'd':
status = 'turn right'
# stop
elif key == 'f':
status = 'stop'
# move
move(status, speed)
# take photo
elif key == 't':
take_photo()
# quit
elif key == readchar.key.CTRL_C:
print('\nquit ...')
px.stop()
Vilib.camera_close()
break
sleep(0.1)
if __name__ == "__main__":
try:
main()
except Exception as e:
print("error:%s"%e)
finally:
px.stop()
Vilib.camera_close()