2.1.3 Tilt Switch¶
Introduction¶
This is a ball tilt-switch with a metal ball inside. It is used to detect inclinations of a small angle.
Required Components¶
In this project, we need the following components.
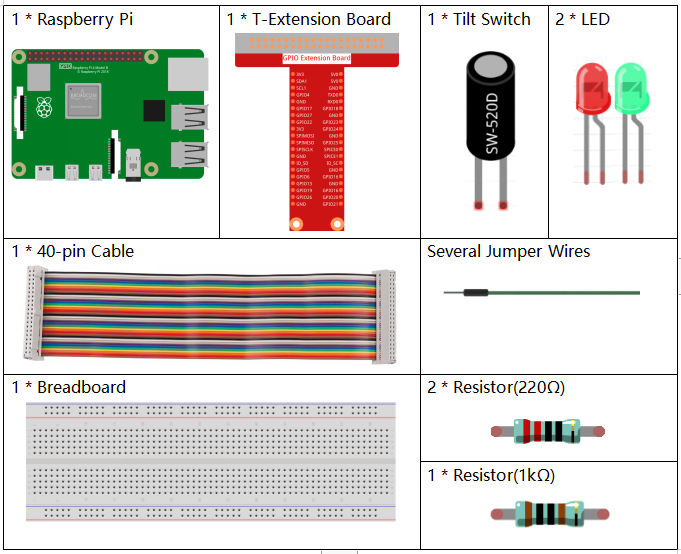
Schematic Diagram¶
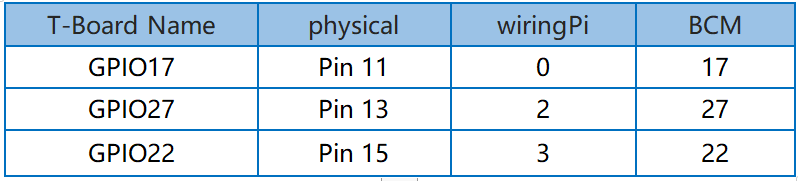
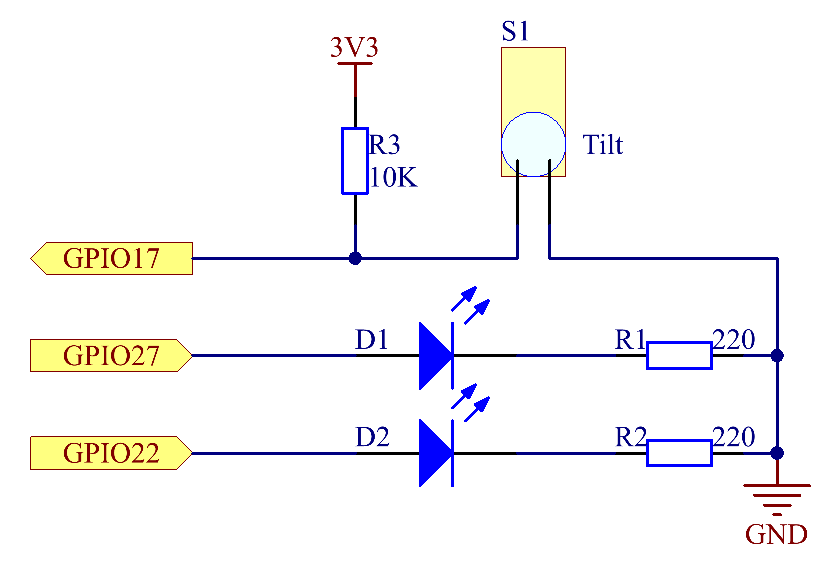
Experimental Procedures¶
Step 1: Build the circuit.
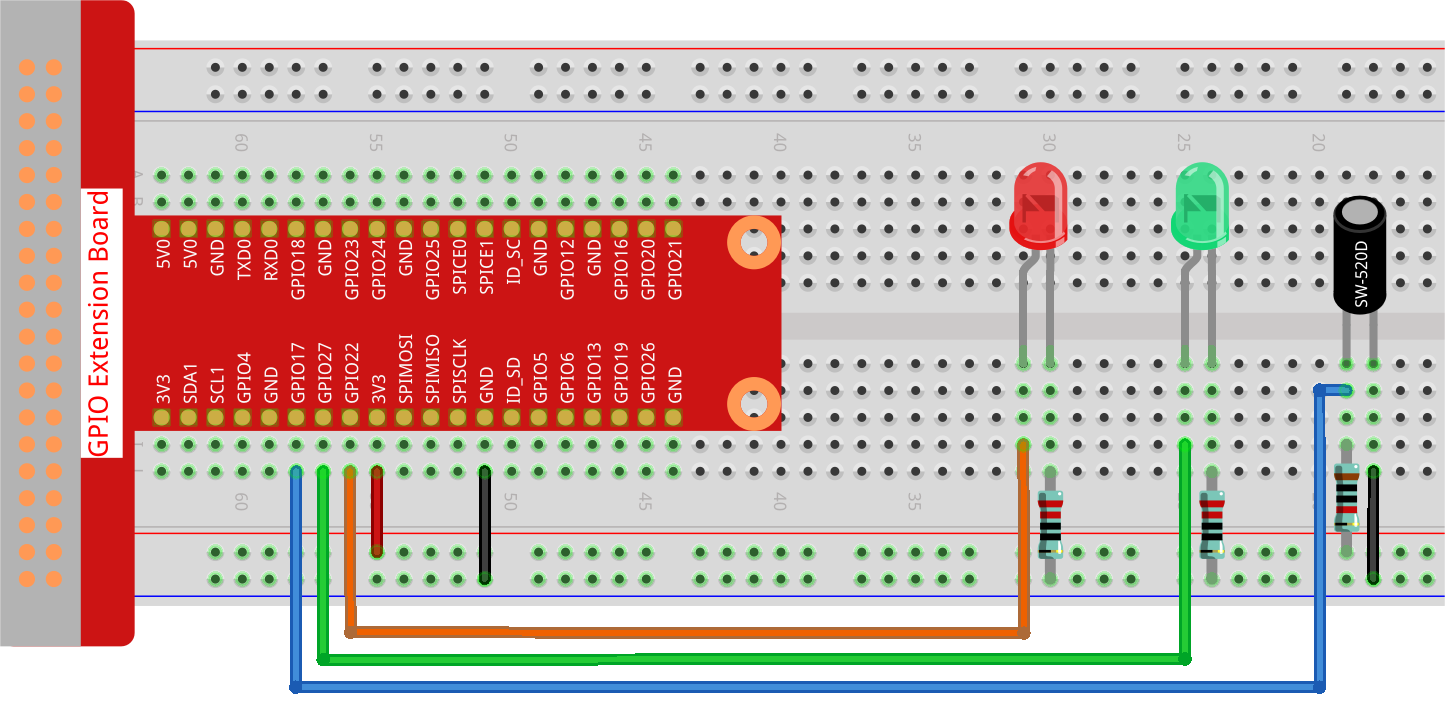
Step 2: Change directory.
cd ~/davinci-kit-for-raspberry-pi/python-pi5
Step 3: Run.
sudo python3 2.1.3_Tilt_zero.py
Place the tilt vertically, and the green LED will turns on. If you tilt it, “Tilt!” will be printed on the screen and the red LED will turns on. Place it vertically again, and the green LED will lights on.
Code
Note
You can Modify/Reset/Copy/Run/Stop the code below. But before that, you need to go to source code path like davinci-kit-for-raspberry-pi/python-pi5
. After modifying the code, you can run it directly to see the effect.
#!/usr/bin/env python3
from gpiozero import LED, Button
# Initialize the Button for the tilt sensor and LEDs using GPIO Zero
TiltPin = Button(17, pull_up=False) # Tilt sensor connected to GPIO pin 17, pull-up resistor disabled
green_led = LED(27) # Green LED connected to GPIO pin 27
red_led = LED(22) # Red LED connected to GPIO pin 22
def detect():
"""
Detect the tilt sensor state and control the LEDs.
Turns on the red LED and turns off the green LED when tilted.
Turns off the red LED and turns on the green LED when not tilted.
"""
if TiltPin.is_pressed: # Check if the sensor is tilted
print(' *************')
print(' * Tilt! *')
print(' *************')
red_led.on() # Turn on red LED
green_led.off() # Turn off green LED
else: # If the sensor is not tilted
red_led.off() # Turn off red LED
green_led.on() # Turn on green LED
try:
while True:
# Continuously check the tilt sensor state and update LEDs
TiltPin.when_pressed = detect
TiltPin.when_released = detect
except KeyboardInterrupt:
# Handle KeyboardInterrupt (Ctrl+C) to exit the loop gracefully
pass
Code Explanation
This line sets the script to run with Python 3 and imports
LED
andButton
fromgpiozero
for controlling GPIO devices.#!/usr/bin/env python3 from gpiozero import LED, Button
Initializes the tilt sensor connected to GPIO pin 17 (with pull-up resistor disabled) and two LEDs connected to GPIO pins 27 and 22.
# Initialize the Button for the tilt sensor and LEDs using GPIO Zero TiltPin = Button(17, pull_up=False) # Tilt sensor connected to GPIO pin 17, pull-up resistor disabled green_led = LED(27) # Green LED connected to GPIO pin 27 red_led = LED(22) # Red LED connected to GPIO pin 22
Defines the
detect
function, which checks the state of the tilt sensor. If tilted, it turns on the red LED and off the green LED. If not tilted, it does the opposite.def detect(): """ Detect the tilt sensor state and control the LEDs. Turns on the red LED and turns off the green LED when tilted. Turns off the red LED and turns on the green LED when not tilted. """ if TiltPin.is_pressed: # Check if the sensor is tilted print(' *************') print(' * Tilt! *') print(' *************') red_led.on() # Turn on red LED green_led.off() # Turn off green LED else: # If the sensor is not tilted red_led.off() # Turn off red LED green_led.on() # Turn on green LED
The main loop assigns the
detect
function to bothwhen_pressed
andwhen_released
events of the tilt sensor. Thetry-except
block handles a KeyboardInterrupt for graceful termination.try: while True: # Continuously check the tilt sensor state and update LEDs TiltPin.when_pressed = detect TiltPin.when_released = detect except KeyboardInterrupt: # Handle KeyboardInterrupt (Ctrl+C) to exit the loop gracefully pass