Passive Buzzer Module¶
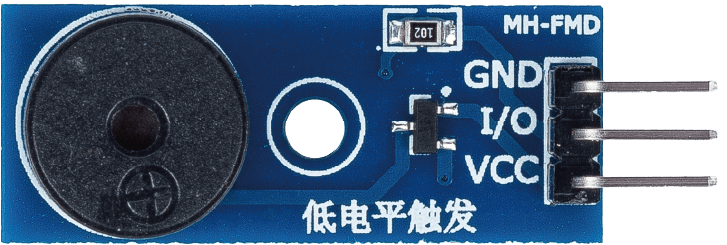
Introduction¶
A passive buzzer is a device that generates sound when an electrical signal is applied to it. It is called passive because it does not have an internal oscillator to generate sound on its own. Instead, it relies on an external signal from a microcontroller like Arduino to produce sound. The passive buzzer module is a small electronic component that contains a passive buzzer and some additional circuitry that makes it easier to use with Arduino.
Principle¶
The working principle of the passive buzzer module is based on the piezoelectric effect. When an electrical signal is applied to the buzzer, it causes a piezoelectric crystal inside the buzzer to vibrate at a specific frequency. This vibration produces sound waves that we can hear. The frequency of the sound produced by the buzzer depends on the frequency of the electrical signal applied to it. By changing the frequency of the signal, we can change the pitch of the sound produced by the buzzer.
Usage¶
Hardware components
Arduino Uno R4 or R3 board * 1
Passive Buzzer Module * 1
Jumper Wires
Circuit Assembly

Code¶
Code explanation¶
Including the pitches library: This library provides the frequency values for various musical notes, allowing you to use musical notation in your code.
#include "pitches.h"
Defining constants and arrays:
buzzerPin
is the digital pin on the Arduino where the buzzer is connected.melody[]
is an array that stores the sequence of notes to be played.noteDurations[]
is an array that stores the duration of each note in the melody.
const int buzzerPin = 8; int melody[] = { NOTE_C4, NOTE_G3, NOTE_G3, NOTE_A3, NOTE_G3, 0, NOTE_B3, NOTE_C4 }; int noteDurations[] = { 4, 8, 8, 4, 4, 4, 4, 4 };
Playing the melody:
The
for
loop iterates over each note in the melody.The
tone()
function plays a note on the buzzer for a specific duration.A delay is added between notes to distinguish them.
The
noTone()
function stops the sound.
void setup() { for (int thisNote = 0; thisNote < 8; thisNote++) { int noteDuration = 1000 / noteDurations[thisNote]; tone(buzzerPin, melody[thisNote], noteDuration); int pauseBetweenNotes = noteDuration * 1.30; delay(pauseBetweenNotes); noTone(buzzerPin); } }
Empty loop function: Since the melody is played only once in the setup, there’s no code in the loop function.
Additional Ideas¶
Modify the melody: You can experiment by changing the notes and durations in the
melody[]
andnoteDurations[]
arrays to create your own tunes. If you are interested, there is a repository (robsoncouto/arduino-songs ) on GitHub that provides Arduino code for playing different songs. Although their approach may be different from this project, you can refer to their notes and durations.Add a button: Integrate a push-button to the circuit and modify the code to play the melody when the button is pressed.