2.2.2 Thermistor¶
Introduction¶
Just like photoresistor can sense light, thermistor is a temperature sensitive electronic device that can be used for realizing functions of temperature control, such as making a heat alarm.
Required Components¶
In this project, we need the following components.
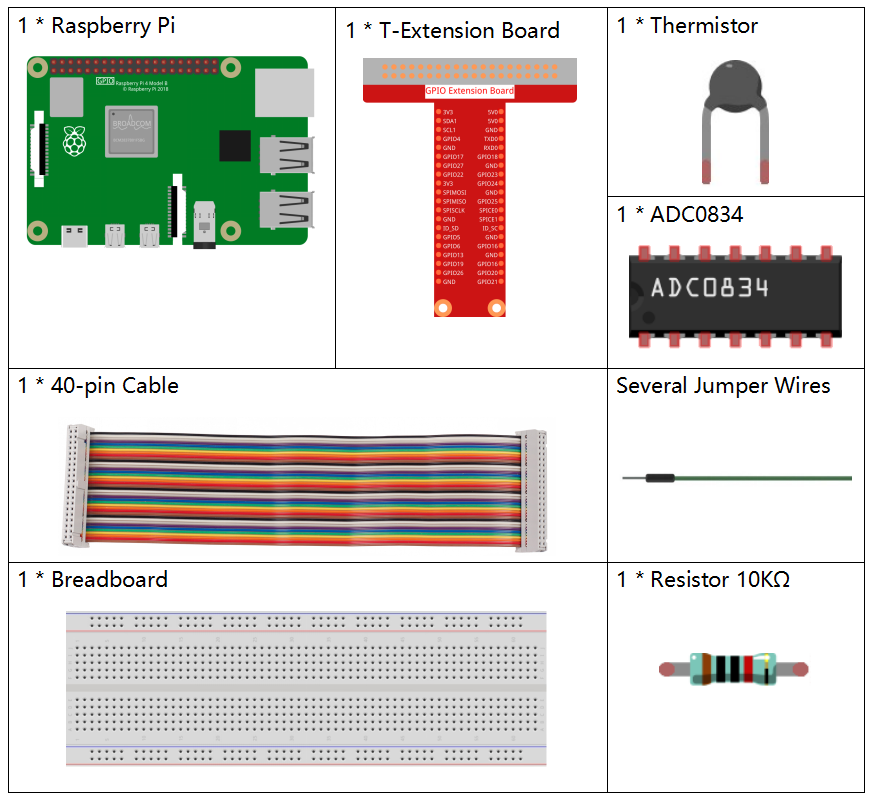
Schematic Diagram¶
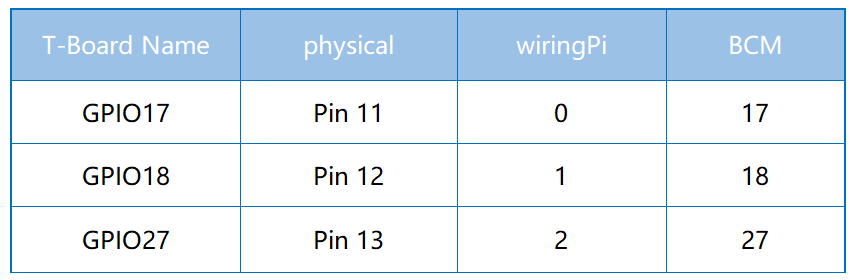
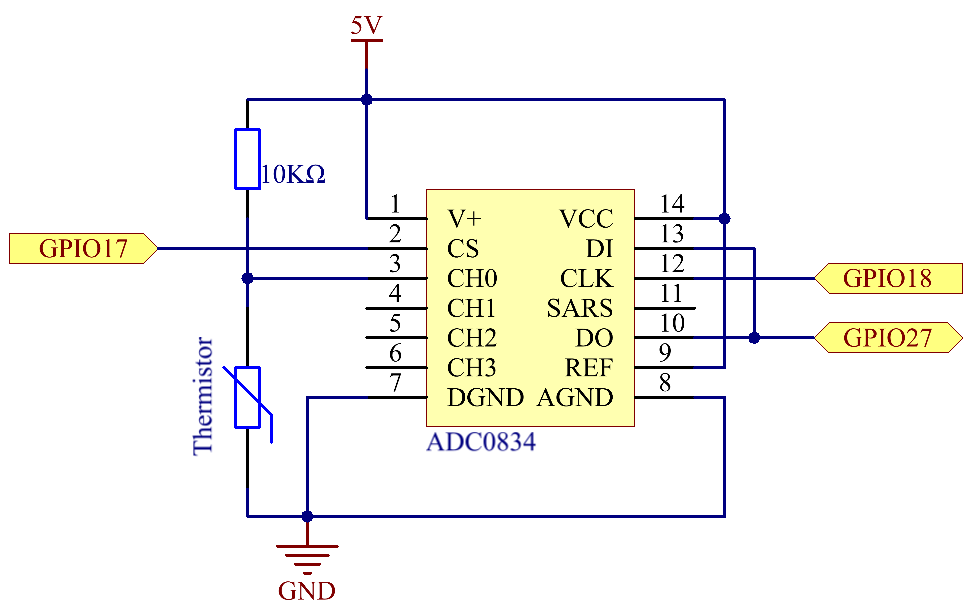
Experimental Procedures¶
Step 1: Build the circuit.
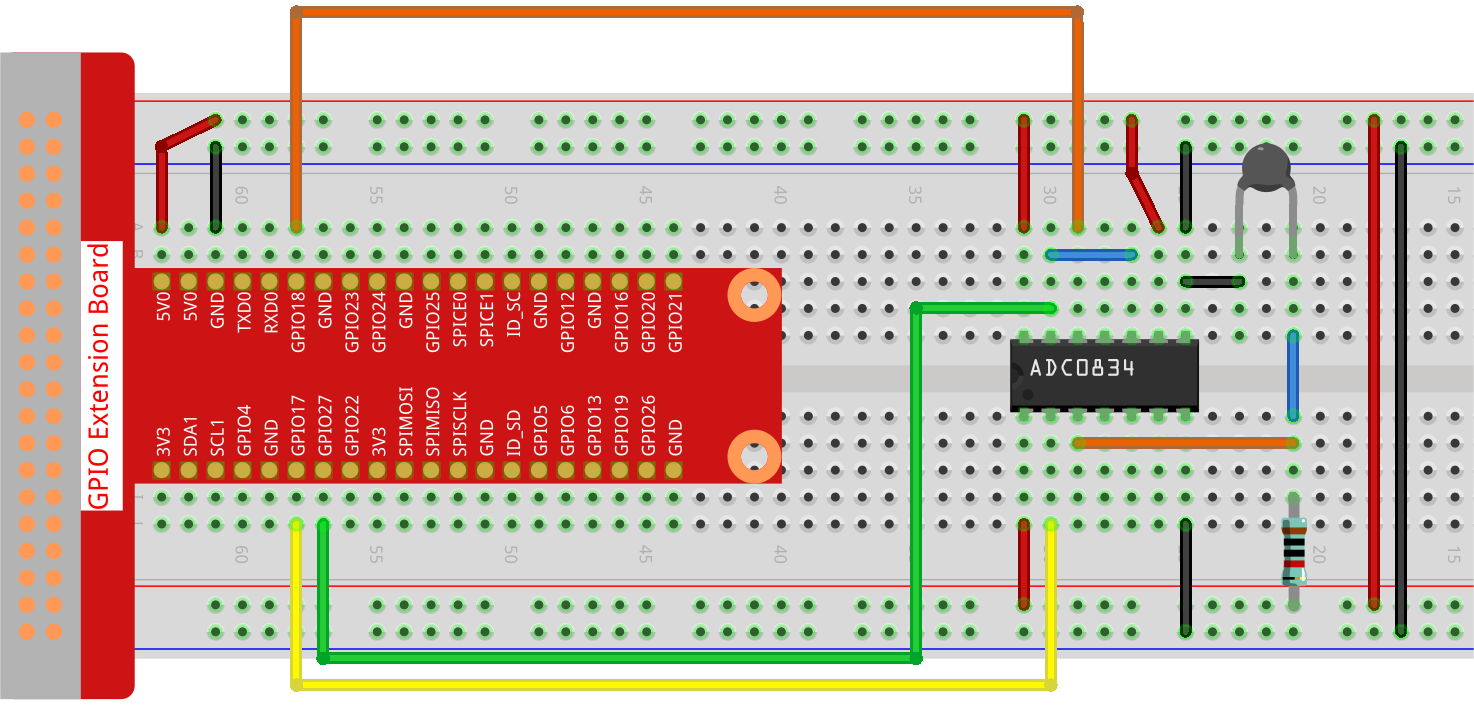
Step 2: Go to the folder of the code.
cd ~/raphael-kit/python-pi5
Step 3: Run the executable file
sudo python3 2.2.2_Thermistor_zero.py
With the code run, the thermistor detects ambient temperature which will be printed on the screen once it finishes the program calculation.
Code
Note
You can Modify/Reset/Copy/Run/Stop the code below. But before that, you need to go to source code path like raphael-kit/python-pi5
. After modifying the code, you can run it directly to see the effect.
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
import ADC0834
import time
import math
# Initialize the ADC0834 module
ADC0834.setup()
# Run the process in a try-except block
try:
while True:
# Read the analog value from the sensor
analogVal = ADC0834.getResult()
# Convert the analog value to a voltage
Vr = 5 * float(analogVal) / 255
# Calculate the resistance of the thermistor
Rt = 10000 * Vr / (5 - Vr)
# Calculate the temperature in Kelvin
temp = 1 / (((math.log(Rt / 10000)) / 3950) + (1 / (273.15 + 25)))
# Convert Kelvin to Celsius
Cel = temp - 273.15
# Convert Celsius to Fahrenheit
Fah = Cel * 1.8 + 32
# Print the temperature in both Celsius and Fahrenheit
print('Celsius: %.2f C Fahrenheit: %.2f F' % (Cel, Fah))
# Wait for 0.2 seconds before the next read
time.sleep(0.2)
# Handle KeyboardInterrupt for graceful termination
except KeyboardInterrupt:
# Clean up ADC0834 resources
ADC0834.destroy()
Code Explanation
This section imports the ADC0834 library for analog-to-digital conversion, the time library for implementing delays, and the math library for conducting mathematical operations.
#!/usr/bin/env python3 # -*- coding: utf-8 -*- import ADC0834 import time import math
Initializes the ADC0834 module to enable reading of analog values.
# Initialize the ADC0834 module ADC0834.setup()
Implements an infinite loop for continuous data reading. The loop reads the analog value from a thermistor, converts this value to voltage, calculates the thermistor’s resistance, and then translates this resistance into temperature measurements in Kelvin, Celsius, and Fahrenheit. It also outputs the temperature readings in Celsius and Fahrenheit, pausing for 0.2 seconds between each reading.
# Run the process in a try-except block try: while True: # Read the analog value from the sensor analogVal = ADC0834.getResult() # Convert the analog value to a voltage Vr = 5 * float(analogVal) / 255 # Calculate the resistance of the thermistor Rt = 10000 * Vr / (5 - Vr) # Calculate the temperature in Kelvin temp = 1 / (((math.log(Rt / 10000)) / 3950) + (1 / (273.15 + 25))) # Convert Kelvin to Celsius Cel = temp - 273.15 # Convert Celsius to Fahrenheit Fah = Cel * 1.8 + 32 # Print the temperature in both Celsius and Fahrenheit print('Celsius: %.2f C Fahrenheit: %.2f F' % (Cel, Fah)) # Wait for 0.2 seconds before the next read time.sleep(0.2)
Catches a KeyboardInterrupt exception to gracefully terminate the program and includes clean-up instructions for the ADC0834 resources upon termination.
# Handle KeyboardInterrupt for graceful termination except KeyboardInterrupt: # Clean up ADC0834 resources ADC0834.destroy()