6.5 Reaction Game¶
Our body has many reaction times, such as audio RT, visual RT, touch RT, etc.
Reaction times have many effects on our daily life, for example, slower than normal reaction times when driving can lead to serious consequences.
In this project, we use 3 buttons and 2 LEDs to measure our visual reaction time.
The serial monitor of the Arduino displays the message “waiting…” After pressing the Ready button, one of the two LEDs must light up randomly after a random time interval. It is important that the testee pushes the corresponding button as soon as possible. The Arduino records the time difference between when the LED lights up and when the person presses the corresponding button, and prints the measured response time on the Arduino serial monitor.
Required Components
In this project, we need the following components.
It’s definitely convenient to buy a whole kit, here’s the link:
Name |
ITEMS IN THIS KIT |
LINK |
---|---|---|
3 in 1 Starter Kit |
380+ |
You can also buy them separately from the links below.
COMPONENT INTRODUCTION |
PURCHASE LINK |
---|---|
Schematic
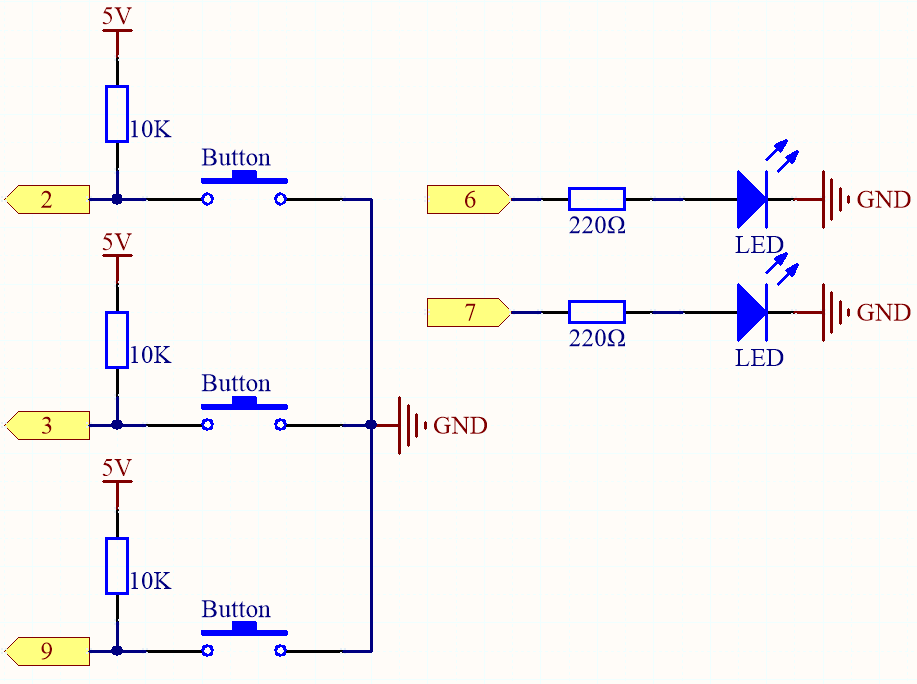
Wiring
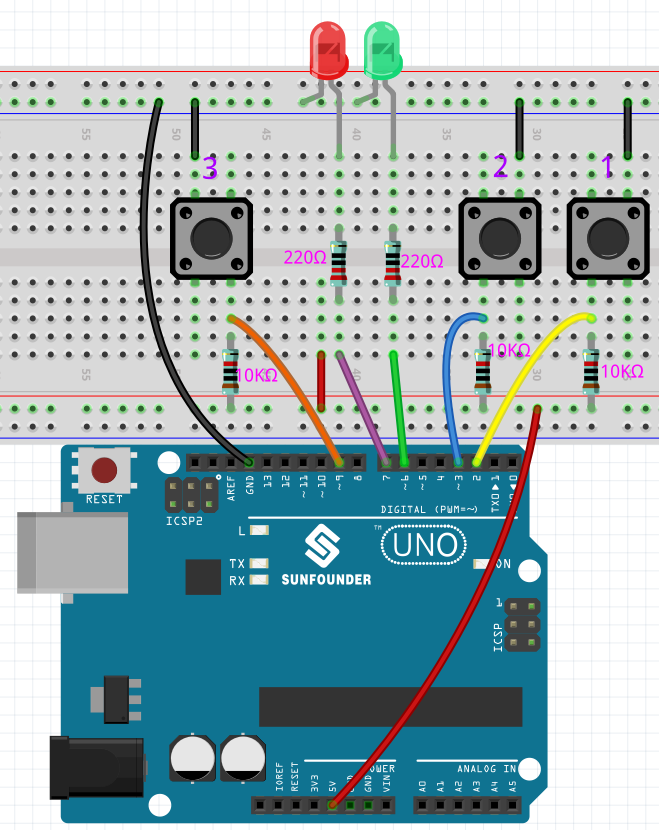
Code
Note
You can open the file
6.5_reaction_time.ino
under the path of3in1-kit\basic_project\6.5_reversingAid
directly.Or copy this code into Arduino IDE 1/2.
Please make sure you have added the
LiquidCrystal_I2C
library, detailed tutorials refer to 5.11 Install External Libraries.
How it works?
Initialize the buttons and LEDs, 2 interrupt are used here to read the button status.
void setup() { ... attachInterrupt(digitalPinToInterrupt(buttonPin1), pressed1, FALLING); attachInterrupt(digitalPinToInterrupt(buttonPin2), pressed2, FALLING); ... }
If the
rstBtn
button is pressed, the game starts again. At a random time between 2 and 5ms, make one of the LEDs light up.void loop() { if (flag == -1 && digitalRead(rstBtn) == LOW) { digitalWrite(ledPin1, LOW); digitalWrite(ledPin2, LOW); Serial.println("Waiting..."); int randomTime = random(2000, 5000); delay(randomTime); timer = millis(); flag = randomTime % 2; Serial.println("Light!"); if (flag == 0) { digitalWrite(ledPin1, HIGH); } else if (flag == 1) { digitalWrite(ledPin2, HIGH); } } delay(200); }
When flag is -1 and
rstBtn
button is pressed, userandom()
function to generate a random time of 2-5s.This time is then used to control the lighting of the LEDs.
Also the lighting of 2 LEDs is randomly generated by
randomTime % 2
with 0 and 1. If flag is 0, then LED1 is lit; if 1, then LED2 is lit.
About
pressed1()
functionvoid pressed1() { if (flag == -1) { return; } if (flag == 0) { int currentTime = millis(); Serial.print("Correct! You reaction time is : "); Serial.print(currentTime - timer); Serial.println(" ms"); } else if (flag == 1) { Serial.println("Wrong Click!"); } flag = -1; }
This is the function that will be triggered when button 1 is pressed. When button 1 is pressed, if the flag is 0 at this time, the response time will be printed, otherwise the press error will be prompted.
About
pressed2()
functionvoid pressed2() { if (flag == -1) { return; } if (flag == 1) { int currentTime =millis(); Serial.print("Correct! You reaction time is : "); Serial.print(currentTime - timer); Serial.println(" ms"); } else if (flag == 0) { Serial.println("Wrong Click!"); } flag = -1; }
This is the function that will be triggered when button 2 is pressed. When button 2 is pressed, if the flag is 1 at this time, the response time will be printed, otherwise the press error will be prompted.